Image Generators¶
AudiverisOmrImageGenerator
Module¶
- AudiverisOmrImageGenerator.extract_symbols(raw_data_directory: str, destination_directory: str)[source]¶
Extracts the symbols from the raw XML documents and matching images of the Audiveris OMR dataset into individual symbols
- Parameters:
raw_data_directory – The directory, that contains the xml-files and matching images
destination_directory – The directory, in which the symbols should be generated into. One sub-folder per symbol category will be generated automatically
CapitanImageGenerator
Module¶
- CapitanImageGenerator.create_capitan_images(raw_data_directory: str, destination_directory: str, stroke_thicknesses: List[int]) None [source]¶
Creates a visual representation of the Capitan strokes by parsing all text-files and the symbols as specified by the parameters by drawing lines that connect the points from each stroke of each symbol.
- Parameters:
raw_data_directory – The directory, that contains the raw capitan dataset
destination_directory – The directory, in which the symbols should be generated into. One sub-folder per symbol category will be generated automatically
stroke_thicknesses – The thickness of the pen, used for drawing the lines in pixels. If multiple are specified, multiple images will be generated that have a different suffix, e.g. 1-16-3.png for the 3-px version and 1-16-2.png for the 2-px version of the image 1-16
HomusImageGenerator
Module¶
- static HomusImageGenerator.create_images(raw_data_directory: str, destination_directory: str, stroke_thicknesses: List[int], canvas_width: int = None, canvas_height: int = None, staff_line_spacing: int = 14, staff_line_vertical_offsets: List[int] = None, random_position_on_canvas: bool = False) dict [source]¶
Creates a visual representation of the Homus Dataset by parsing all text-files and the symbols as specified by the parameters by drawing lines that connect the points from each stroke of each symbol.
Each symbol will be drawn in the center of a fixed canvas, specified by width and height.
- Parameters:
raw_data_directory – The directory, that contains the text-files that contain the textual representation of the music symbols
destination_directory – The directory, in which the symbols should be generated into. One sub-folder per symbol category will be generated automatically
stroke_thicknesses – The thickness of the pen, used for drawing the lines in pixels. If multiple are specified, multiple images will be generated that have a different suffix, e.g. 1-16-3.png for the 3-px version and 1-16-2.png for the 2-px version of the image 1-16
canvas_width – The width of the canvas, that each image will be drawn upon, regardless of the original size of the symbol. Larger symbols will be cropped. If the original size of the symbol should be used, provided None here.
canvas_height – The height of the canvas, that each image will be drawn upon, regardless of the original size of the symbol. Larger symbols will be cropped. If the original size of the symbol should be used, provided None here
staff_line_spacing – Number of pixels spacing between each of the five staff-lines
staff_line_vertical_offsets – List of vertical offsets, where the staff-lines will be superimposed over the drawn images. If None is provided, no staff-lines will be superimposed. If multiple values are provided, multiple versions of each symbol will be generated with the appropriate staff-lines, e.g. 1-5_3_offset_70.png and 1-5_3_offset_77.png for two versions of the symbol 1-5 with stroke thickness 3 and staff-line offsets 70 and 77 pixels from the top.
random_position_on_canvas – True, if the symbols should be randomly placed on the fixed canvas. False, if the symbols should be centered in the fixed canvas. Note that this flag only has an effect, if fixed canvas sizes are used.
- Returns:
A dictionary that contains the file-names of all generated symbols and the respective bounding-boxes of each symbol.
HomusSymbol
Module¶
- class omrdatasettools.HomusImageGenerator.HomusSymbol(content: str, strokes: List[List[Point2D]], symbol_class: str, dimensions: Rectangle)[source]¶
- static HomusSymbol.initialize_from_string(content: str) HomusSymbol [source]¶
Create and initializes a new symbol from a string
- Parameters:
content – The content of a symbol as read from the text-file
- Returns:
The initialized symbol
- Return type:
- HomusSymbol.draw_into_bitmap(export_path: ExportPath, stroke_thickness: int, margin: int = 0) None [source]¶
Draws the symbol in the original size that it has plus an optional margin
- Parameters:
export_path – The path, where the symbols should be created on disk
stroke_thickness – Pen-thickness for drawing the symbol in pixels
margin – An optional margin for each symbol
- HomusSymbol.draw_onto_canvas(export_path: ExportPath, stroke_thickness: int, margin: int, destination_width: int, destination_height: int, staff_line_spacing: int = 14, staff_line_vertical_offsets: List[int] = None, bounding_boxes: dict = None, random_position_on_canvas: bool = False) None [source]¶
Draws the symbol onto a canvas with a fixed size
- Parameters:
bounding_boxes – The dictionary into which the bounding-boxes will be added of each generated image
export_path – The path, where the symbols should be created on disk
stroke_thickness –
margin –
destination_width –
destination_height –
staff_line_spacing –
staff_line_vertical_offsets – Offsets used for drawing staff-lines. If None provided, no staff-lines will be drawn if multiple integers are provided, multiple images will be generated
MeasureVisualizer
Module¶
This class can be used to generate visualizations of measure annotations, such as this one for the Muscima++ dataset:
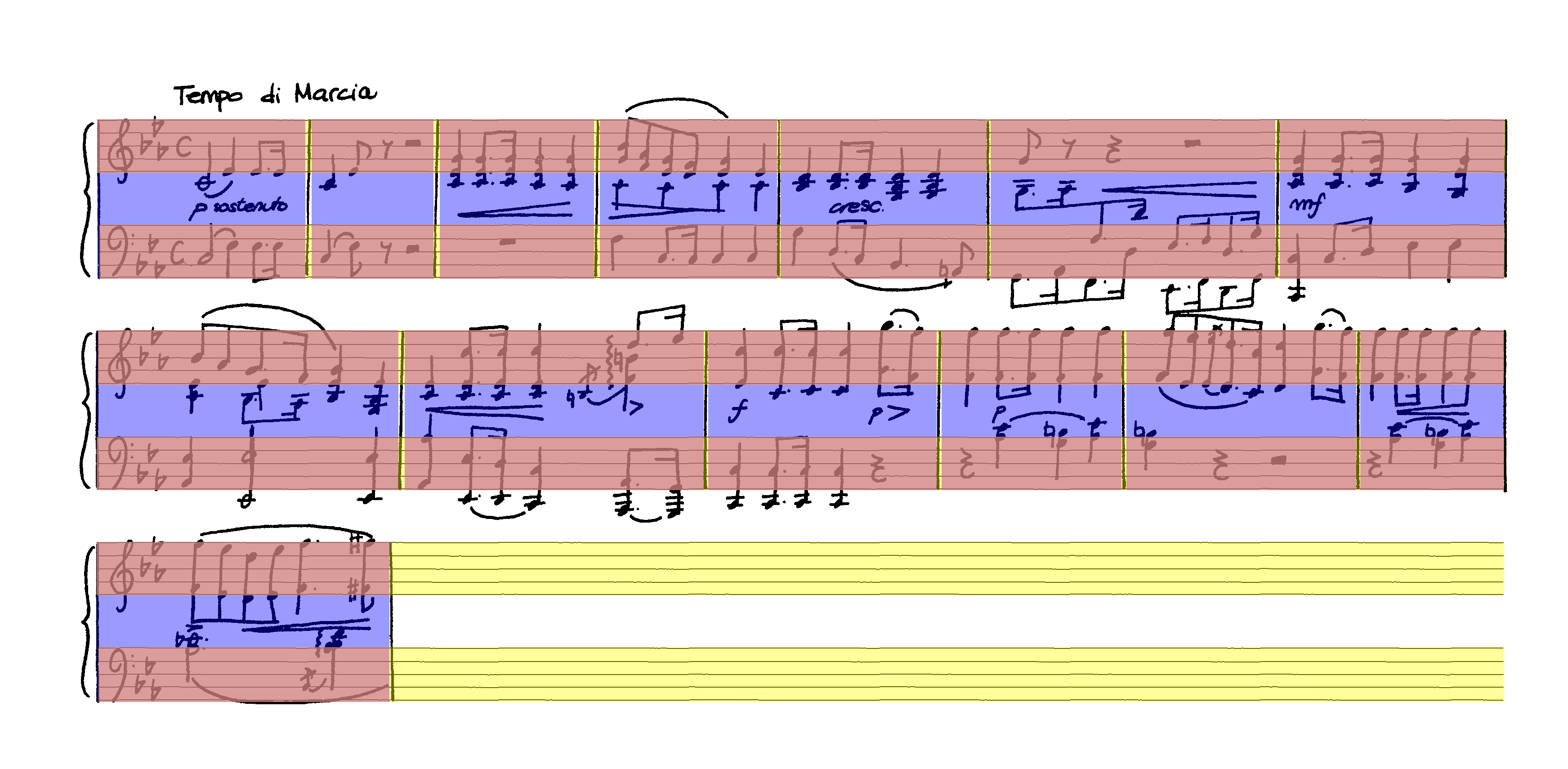
- class omrdatasettools.MeasureVisualizer.MeasureVisualizer(draw_system_measures: bool, draw_stave_measures: bool, draw_staves: bool)[source]¶
Class that can be used to visualize the measure annotations that are provided as json files. Allows to enable/disable whether to draw system-measures (one measure for all instruments), stave-measures (one measure, single stave of one instrument) and staves (the whole line of a single instrument). System-measures contain all stave-measures that are being played simulatenously.
Bounding boxes will be drawn with semitransparent colors.
MuscimaPlusPlusMaskImageGenerator
Module¶
- class omrdatasettools.MuscimaPlusPlusMaskImageGenerator.MaskType(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)[source]¶
The type of masks that should be generated
- NODES_SEMANTIC_SEGMENTATION = 1¶
creates mask images, where each type of node gets the same color mask (for semantic segmentation). The classes staffLine, staff and staffSpace are ignored.
- STAFF_BLOBS_INSTANCE_SEGMENTATION = 3¶
creates mask images, where each staff will receive one big blob (filling the staff space regions) per staff line for instance segmentation. So each staff will have a different color.
- STAFF_LINES_INSTANCE_SEGMENTATION = 2¶
creates mask images, where the masks of the staff lines are contained for instance segmentation. All five lines that form a staff will have the same color.
- MuscimaPlusPlusMaskImageGenerator.render_node_masks(raw_data_directory: str, destination_directory: str, mask_type: MaskType)[source]¶
Extracts all symbols from the raw XML documents and generates individual symbols from the masks
- Parameters:
raw_data_directory – The directory, that contains the xml-files and matching images
destination_directory – The directory, in which the symbols should be generated into. Per file, one mask will be generated.
mask_type – The type of masks that you want to generate, e.g., masks for each node or staff lines only.
MuscimaPlusPlusSymbolImageGenerator
Module¶
- class omrdatasettools.MuscimaPlusPlusSymbolImageGenerator.MuscimaPlusPlusSymbolImageGenerator[source]¶
- MuscimaPlusPlusSymbolImageGenerator.extract_and_render_all_symbol_masks(raw_data_directory: str, destination_directory: str)[source]¶
Extracts all symbols from the raw XML documents and generates individual symbols from the masks
- Parameters:
raw_data_directory – The directory, that contains the xml-files and matching images
destination_directory – The directory, in which the symbols should be generated into. One sub-folder per symbol category will be generated automatically